Tricks to deal with customized Google Analytics and Google Tag Manager integrations
In the past years, I worked on implementing Google Analytlics and Google Tag Manager in a lot of differences scenearios and almost any kind of integrations. So I'm gonna show you some code snippets that may help you integrating Google Tag Manager and Google Analytics into your libraries in the best way possible.
Checking is Universal Analytics is available
Most integrations I've seen does check for the ga object within the DOM before trying to push something to it. This is really nice, and a check that should be done ALWAYS, but Universal Analytics allows you to change the default name for the "ga" object, so under those situations our code may not work as we're expecting.
Luckly for us, Universal Analytics creates another variable, named window.GoogleAnalyticsObject
, that will allow us to grab the current object name being used by Universal Analytics.
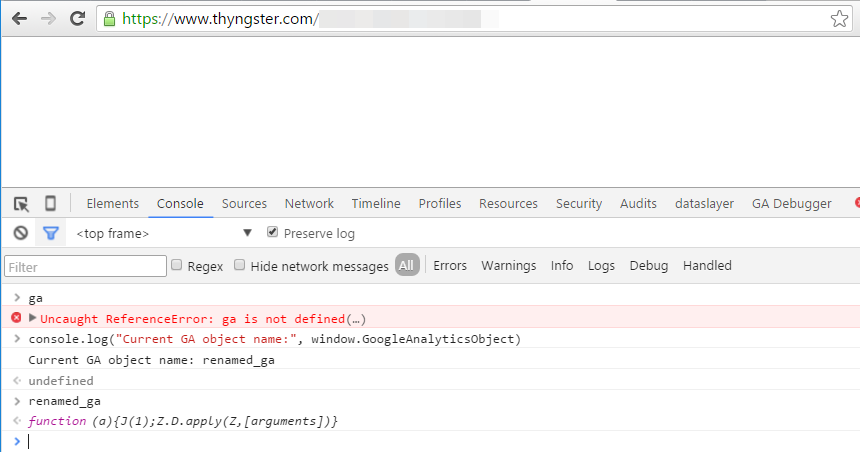
So using the following code will allow us to check is Google Analytics is really available even if the current name has been changed:
if(window.GoogleAnalyticsObject && typeof(window[GoogleAnalyticsObject])=="function"){ // Code Here }
Checking for current dataLayer object name for Google Tag Manager
Google Tag Manager users the "dataLayer" name by default, but it may changed to use any other name.
This piece of code will allow us to find the right dataLayer name dinamically:
var gtm_datalayer_names=[]; if(typeof(google_tag_manager)!="undefined") for(i in google_tag_manager){ if(google_tag_manager[i].gtmDom==true){ gtm_datalayer_names.push(i); } }
The we could do something like:
window[gtm_datalayer_names[0]].push({});
We'll even be able to send pushes to all the different dataLayer available on the current page:
if(gtm_datalayer_names.length>0){ var i = window.gtm_datalayer_names.length; while(i--){ var dlName = gtm_datalayer_names[i]; window[gtm_datalayer_names] = window[gtm_datalayer_names] || []; window[gtm_datalayer_names[i]].push({ 'event': 'my-event-name', 'dataLayerName': gtm_datalayer_names[i] }); } }
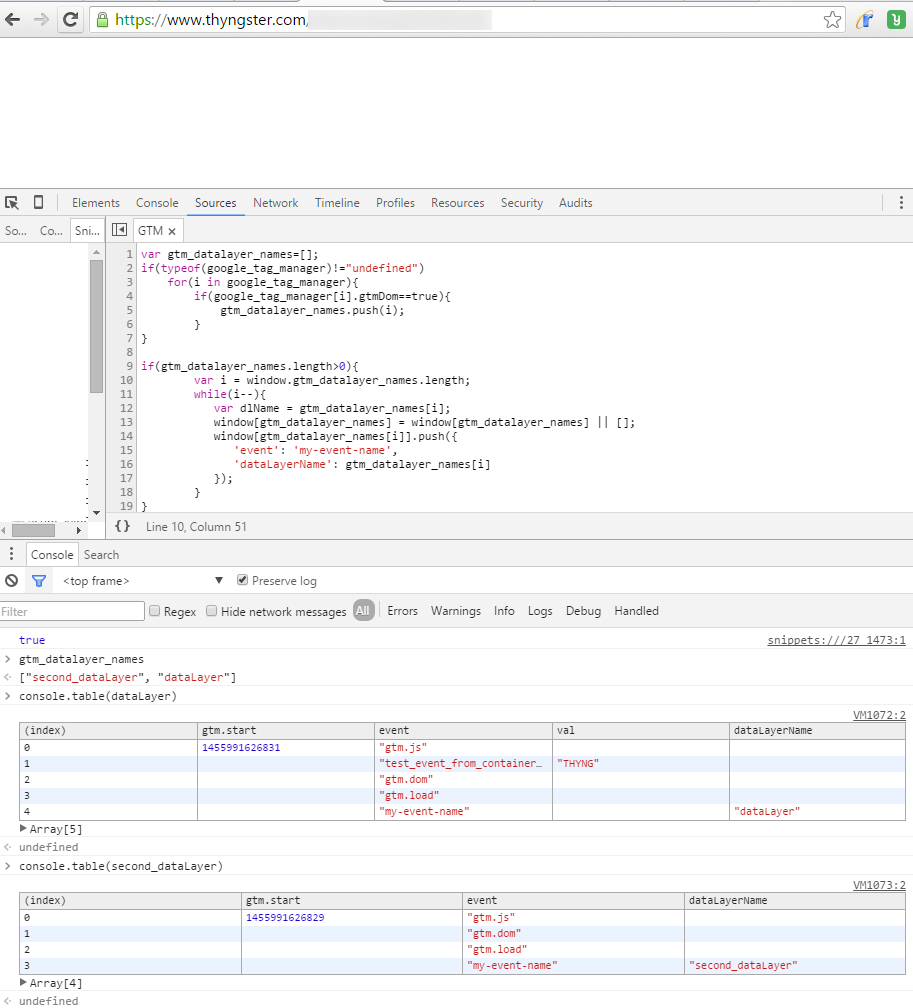